What is C programming ?
C is a popular and widely used programming language that was created in the early 1970s and has since become a standard in computer science. It gives developers a lot of power over their computer's hardware, making it an excellent language for system programming, embedded systems, and operating systems. It also has a straightforward syntax and a variety of capabilities, such as pointers, memory management, and low-level access to computer resources.
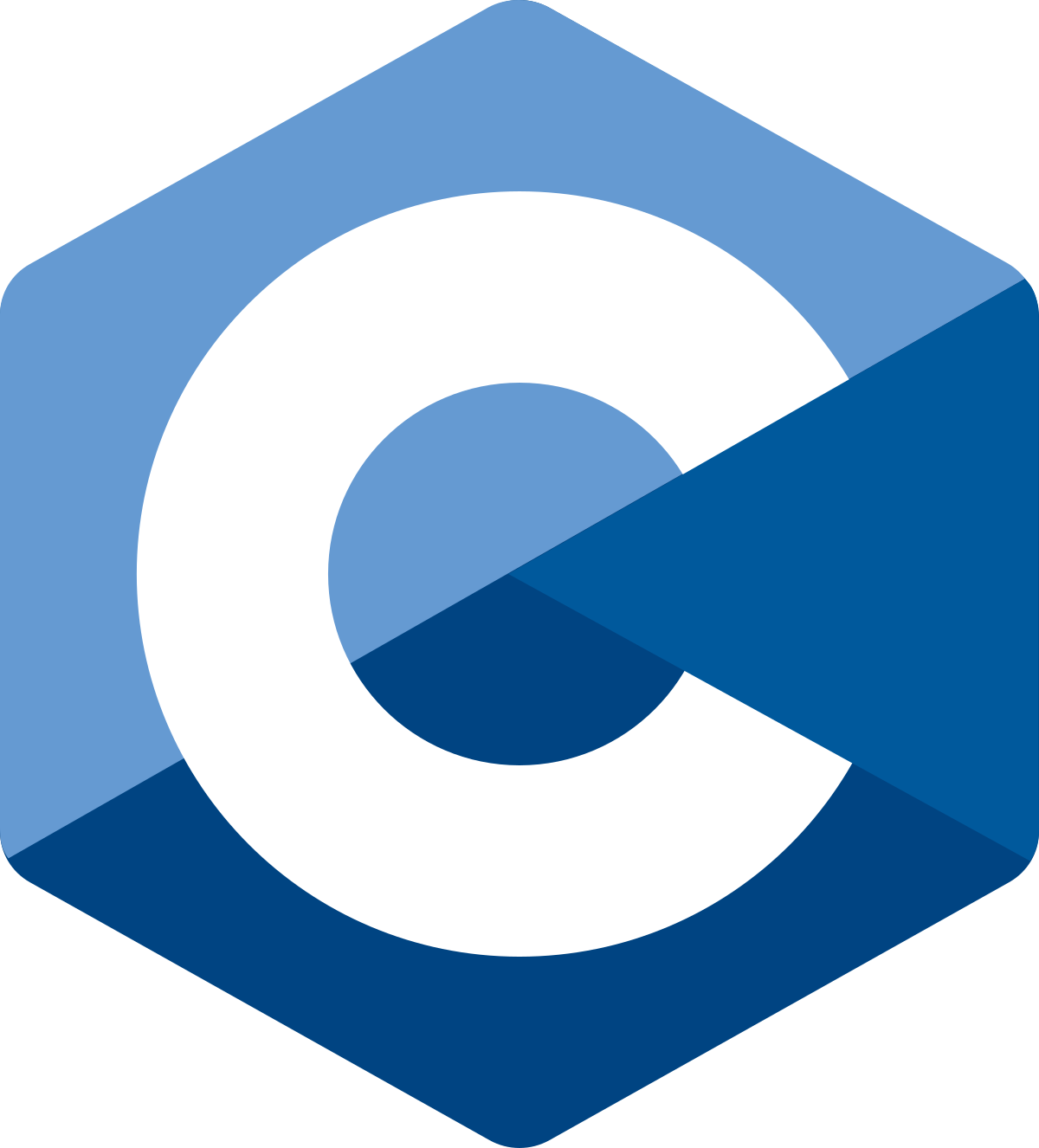
Logo of C Programming
Why is it importance in the world of computer science ?
For various reasons, C programming is an essential language in the realm of computer science:
1.Efficiency
C programming is popular for designing operating systems, device drivers, and embedded systems due to its efficient use of resources.
2.Portability
C programming is a portable language, which means it can be compiled and executed on a wide range of platforms and operating systems.
3.Low level access
C programming is an effective language for system-level programming and hardware control.
4.Widely used
C programming is extensively used in business and academia, and several programming languages are developed on or influenced by it.
5.Great demand
C programmers are in high demand for system programming, embedded systems, and game development.
History of C programming and how it has evolved through time
C programming was developed in the 1970s by Dennis Ritchie at Bell Labs and quickly gained popularity due to its efficiency and low-level code. In 2011, the C11 standard was released, adding new features such as threading support and improved Unicode support. Today, it is a popular language for system-level programming, embedded systems, operating systems, and desktop applications.
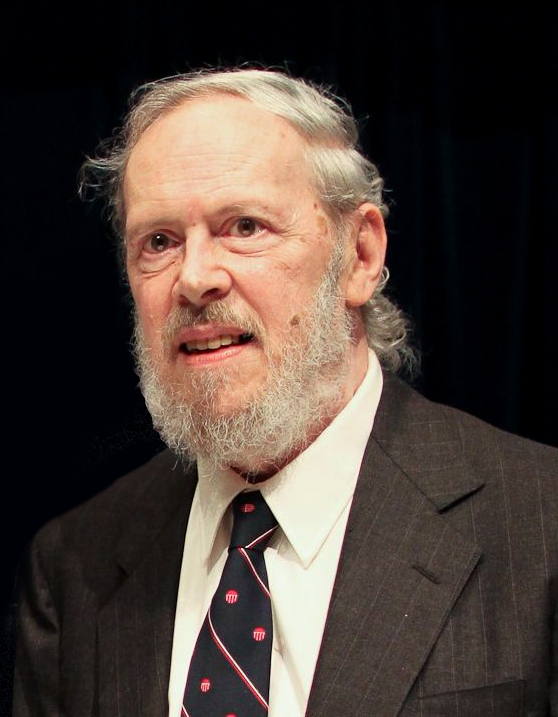
Dennis Ritchie
Why should programmer good at C programming ?
Programmers should be good at C programming for efficiency, low-level access, portability, influence on other languages, and job opportunities. It is portable and can be compiled and executed on a variety of platforms and operating systems. Job opportunities include system programming, embedded systems, and game development.
Tools required to begin coding in C, such as a text editor and a C compiler
A text editor, C compiler, IDE or CLI are necessary for coding in C. A text editor is used to write and edit code, a C compiler translates code into machine-readable code, an IDE combines a text editor, compiler, debugger, and other development tools into a single interface, and a Command Line Interface (CLI) allows users to interact with their computer.
How to configure the development environment on several operating systems (Windows, Mac, and Linux)?
The basic procedures for setting the development environment for C programming on Windows, Mac, and Linux are the same.
1.Windows
Install a C compiler
Get a C compiler, such as GCC or Microsoft Visual C++, and install it. Compiler installation instructions should be followed.
Install a text editor
Get a text editor like Notepad++ or Visual Studio Code and install it.
Set environment variables
Include the directory where the C compiler is installed in the PATH environment variable to launch it from the command prompt.
2.Mac
Xcode to be installed
Xcode is a free programming environment that contains a C compiler. Get Xcode from the Mac App Store and install it.
Install a text editor
Get a text editor like Sublime Text or Visual Studio Code and install it.
Set environment variables
Include the directory where the C compiler is installed in the PATH environment variable. You may now launch the compiler from the terminal.
3.Linux
Install a C compiler
Most Linux distributions include a C compiler. If not, you may install one using the package manager included with your distribution.
Install a text editor
Get a text editor like Atom or Visual Studio Code and install it.
Set environment variables
Include the C compiler's directory in the PATH environment variable and launch it from the terminal. Look for other tools and libraries to improve your C programming environment, such as debuggers and code libraries.
Free and open-source software for C programming
GCC is a popular open-source compiler for C, C++, and other programming languages, available on multiple platforms. It is available on Windows, Mac, and Linux.
Clang
Clang is an open-source C compiler designed to be compatible with GCC, making it a good alternative for C development.
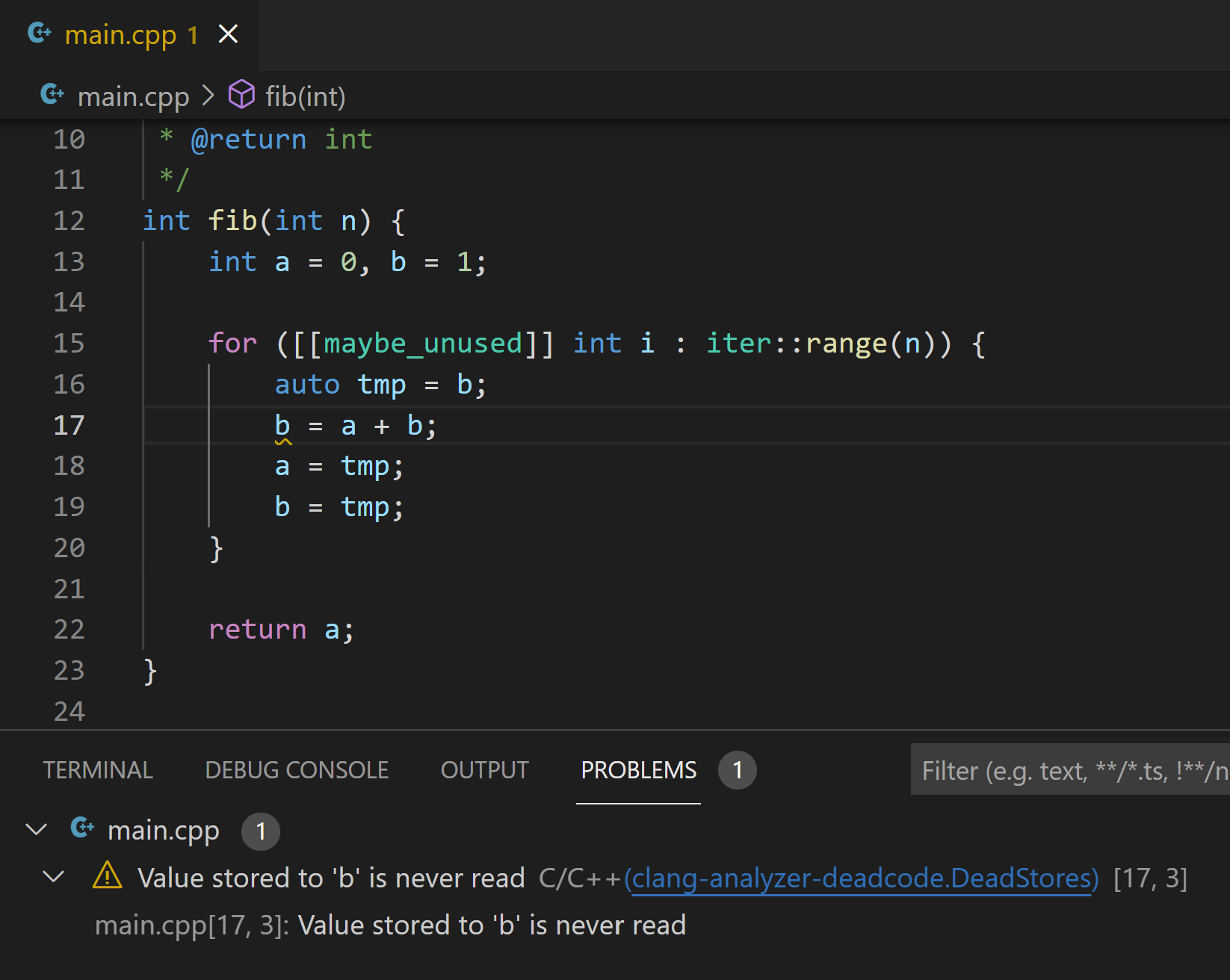
Clang C Programming
Code::Block
Code::Blocks is a free and open-source IDE for C, C++, and Fortran.
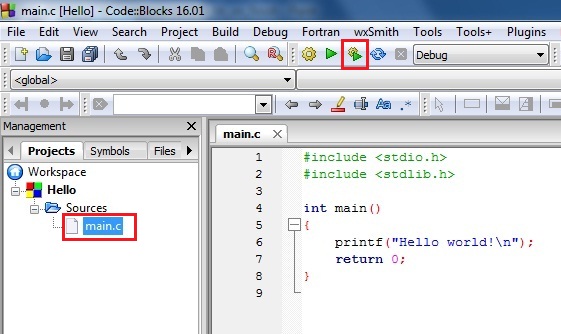
Code::Block c programming
Visual Studio Code
Visual Studio Code is a popular free and open-source code editor that supports C and C++ development.
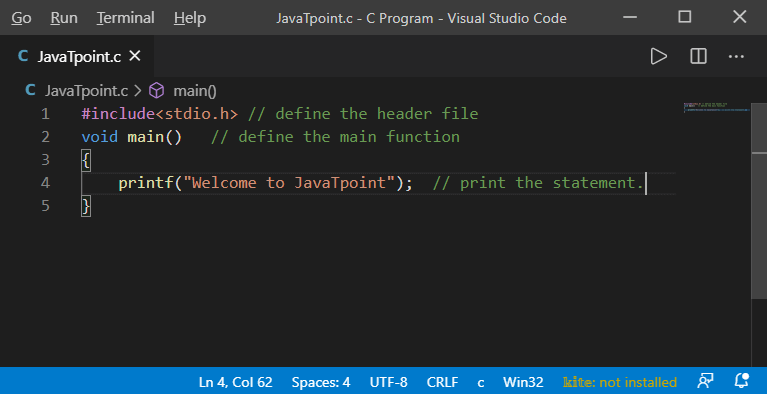
Visual Studio Code C Programming
Eclipse
Eclipse is a free and open-source IDE that supports C and C++ development.
GDB (GNU Debugger)
GDB is a free and open-source debugger that helps programmers and developers write, compile, and debug C programs efficiently and effectively.
Fundamental structure of a C programme, including the main function, curly braces, and semicolons
A C program consists of the main function, curly braces, and semicolons, with an overview of each component.
1. Main function
The main function is the starting point of a C program and is executed when the program is run. It has the following syntax.
```
int main()
{
// Code goes here
return 0;
}
```
The main function has an integer return type and a parentheses to indicate it is a function. The curly braces enclose the code that will be executed when the function is called.
2. Curly braces
Curly braces are used to enclose blocks of code, with the opening brace indicating the start and closing brace indicating the end.
3. Semicolons
Semicolons are used to terminate statements in C, such as to print the text "Hello, World!" to the console.Note that this statement ends with a semicolon.
```
printf("Hello, World!");
```
Data types and variables
Data types and variables, such as integers, floats, and characters, are used to store and manipulate data in C programming. These data types and variables are essential for effective C programs, as they are used to store and manipulate data.
1. Integers
Integers are whole numbers without decimal places, and can be represented using the int data type.
```
int age = 25;
Integers C Programming
2. Floats
Floats are numbers with decimal places, which can be represented using the float or double data type.
```
float height = 1.75;
```
Floats functions in C Programming
3. Characters
Characters can be represented using the char data type, such as the variable firstInitial.
```
char firstInitial = 'J';
Characters functions in C Programming
4. Strings
Strings are a sequence of characters that can be represented using the char data type and an array of characters.
```
char name[] = "John Smith";
```
Strings C programming
5. Booleans
Booleans are true or false values that can be represented using the bool data type.
```
bool isRaining = true;
```
Variables are used to store data values in a program, and must be declared and initialized before use.
```
int age;
float height;
char firstInitial;
age = 25;
height = 1.75;
firstInitial = 'J';
```
```
Booleans functions in C Programming
Creating a basic programme
To create a basic program in C, you will need to follow a few basic steps:
1. Open a text editor
The first step is to open a text editor such as Notepad, Sublime Text, or Visual Studio Code. This is where you will write your program code.
2. Write the code
The main() function is the entry point of a C program, which prints "Hello, World!" to the console.
```
#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
```
#include is a preprocessor directive that includes the printf() function.
3. Save the file
After writing the code, you will need to save the file with a `.c` extension. For example, you could save the file as `hello.c`.
4. Compile the program
Open a terminal or command prompt and run the following command to compile the program.
```
gcc hello.c -o hello
```
This command tells the compiler to compile the `hello.c` file and output the executable program as `hello`.
5. Run the program
Once the program has been compiled, you can run it by entering the following command in the terminal or command prompt:
```
./hello
This will execute the program and print "Hello, World!" to the console.
Deconstruct the programme into smaller jobs
Deconstructing a program into smaller tasks is an important step in software development, such as the "Hello, World!" program.
1. Define the problem:
The first task is to define the problem you are trying to solve. In this case, the problem is to print the text "Hello, World!" to the console.
2. Plan the solution:
The solution to the problem is to write a C program that uses the printf() function to print "Hello, World!" to the console.
3. Write the code:
Break down the solution into smaller steps, such as including libraries, defining the main() function, and using the printf() function.
4. Test the code:
Test the program to ensure it works by running it and printing "Hello, World!" to the console.
5. Debug any issues:
Debugging is the process of identifying and fixing errors in code to ensure the program works as intended.
6. Refactor the code:
Refactoring the code involves reviewing and making any necessary improvements or optimizations to improve the program's performance.
Breaking down a program into smaller tasks can help improve code quality and make the development process more efficient.
How to use expressions, loops, and basic arithmetic in C ?
Expressions, loops, and basic arithmetic are fundamental concepts in C programming. Here is an overview of how to use them:
Expressions
Expressions in C are combinations of values, variables, and operators that can be evaluated to produce a result.
Loops
Loops in C are used to execute a block of code until a certain condition is met, with the for loop printing numbers from 1 to 10.
```
for (int i = 1; i <= 10; i++) {
printf("%d ", i);
}
```
The loop initializes the variable i to 1, executes the loop body while i is less than or equal to 10, and increments it by 1.
3.Basic arithmetic
C supports basic arithmetic operators, such as addition, subtraction, multiplication, division, and modulus.
```
#include <stdio.h>
int main() {
int a = 5;
int b = 3;
int sum = a + b;
int product = a * b;
int quotient = a / b;
int remainder = a % b;
printf("Sum: %d\n", sum);
printf("Product: %d\n", product);
printf("Quotient: %d\n", quotient);
printf("Remainder: %d\n", remainder);
return 0;
}
```
The program uses expressions, loops, and basic arithmetic to compute the sum, product, quotient, and remainder of two variables.
Best practices for writing clean and readable code
Use meaningful variable and function names to make code easier to understand and maintain, and avoid abbreviations and single-letter variable names.
Write comments
Add comments to your code to explain how it works and why it is necessary, making it easier for other developers to understand.
Use indentation and spacing
Use indentation and spacing consistently to make your code easier to read.
3.Break down large functions:
Break down large functions into smaller functions to make them easier to understand and test.
4. Use constants and enumerations:
Use constants and enumerations to make code more readable and easier to modify, and handle errors gracefully to avoid crashes.
5. Use error messages:
Use error messages to inform the user of any problems that occur during execution.
6. Follow a coding standard:
Follow coding standards to ensure code is consistent and easy to read, making it easier to work with in the long run.
How to run the compiled program and test its functionality?
To run a compiled program in C and test its functionality, follow these steps:
1. Compile the program
Use a C compiler to compile your program. For example, if you are using gcc on a Linux system, you can use the following command:
```
gcc -o program program.c
```
This command will compile the program.c source file and create an executable file called program.
2. Run the program
To run the program, use the following command:
```
./program
```
This command will execute the program and display the output on the terminal.
3. Test the functionality
Programs can be tested by providing input values and checking the output, such as calculating the sum of two integers.
4. Debug the program
Use a debugger to find and fix errors in a program, such as setting breakpoints and examining variables.
5. Repeat the process
Modify and run your C program until it works correctly, then use a debugger to identify and fix any errors or bugs.
How to allocate and deallocate memory in C programming?
In C programming, you can allocate and deallocate memory dynamically using pointers and memory allocation functions. Here are the steps to allocate and deallocate memory in C:
1. Allocate memory:
Use the malloc() function to allocate memory dynamically. The malloc() function returns a void pointer to the allocated memory. The syntax for malloc() is:
```
ptr = (cast-type*) malloc(byte-size);
```
where ptr is a pointer to the first byte of the allocated memory block, cast-type is the type to which the pointer is cast, and byte-size is the number of bytes to allocate.
For example, to allocate memory for an integer variable, use the following code:
```
int *ptr;
ptr = (int*) malloc(sizeof(int));
```
This code allocates memory for an integer variable and returns a pointer to the first byte of the allocated memory block.
2. Initialize memory
Use the memset() function to initialize the memory block with a specific value. The syntax for memset() is:
```
memset(ptr, value, size);
```
where ptr is a pointer to the memory block, value is the value to set each byte to, and size is the number of bytes to set.
3. Deallocate memory
Use the free() function to deallocate memory that was previously allocated with malloc(). The syntax for free() is:
```
free(ptr);
```
where ptr is a pointer to the memory block to deallocate.
For example, to deallocate the memory block allocated in the previous example, use the following code:
```
free(ptr);
```
It is important to deallocate memory when it is no longer needed to avoid memory leaks.
Advanced concepts in C programming
C programming is a powerful language with advanced concepts to create complex and efficient programs. These include pointers, structures and unions, file I/O, function pointers, preprocessor directives, and recursion. Pointers store memory addresses, structures and unions create data structures, file I/O provides functions for reading and writing data, function pointers pass functions as arguments, and preprocessor directives modify source code.
C programming is an important skill to learn and should be explored further
C programming is a popular language in computer science and engineering, and learning it can open up career opportunities. It has a rich history and is still widely used today, and can help gain a deeper understanding of important software systems. Learning C programming is a valuable and rewarding skill that can provide many benefits.