Why Python is an ideal choice for beginners ?
Python is an ideal choice for beginners due to its easy to read and write syntax, gentle learning curve, large community and support, extensive documentation, versatility and broad application, abundance of libraries and frameworks, job opportunities and career growth, and career prospects. It has a gentle learning curve, large community and support, extensive documentation, versatility and broad application, abundance of libraries and frameworks, job opportunities and career growth, and career prospects.
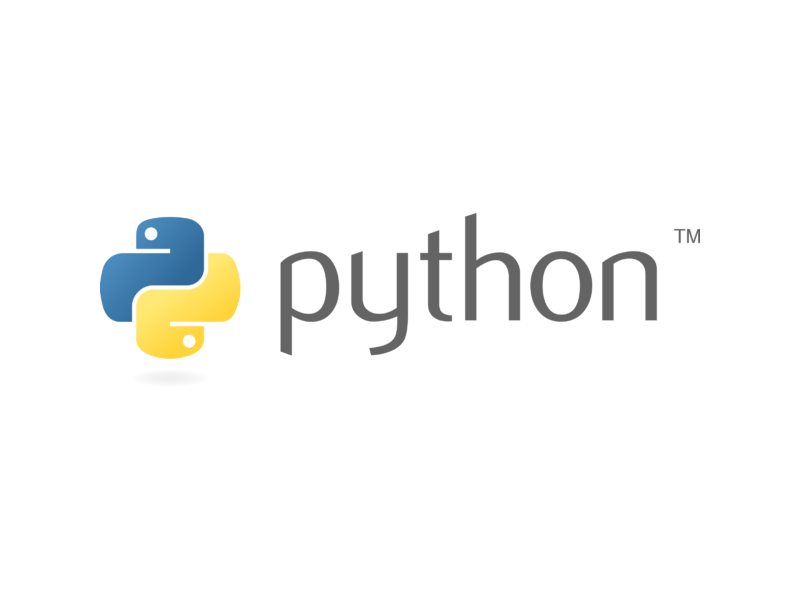
Logo of Phython
Python's popularity and adaptability
Python is a high-level, interpreted programming language created by Guido van Rossum in 1991. It is popular due to its ease of learning, versatility, large and active community, extensive ecosystem, cross-platform compatibility, integration capabilities, job opportunities, and career growth. Its user-friendly nature and extensive ecosystem make it an attractive choice for both beginners and experienced developers.
Getting Started with Python
Getting started with Python is an exciting journey that opens up a world of possibilities in programming.
Here are the essential steps to get you started:
1. Install Python
Start by downloading and installing Python on your computer.
Follow the installation instructions provided.
2.Choose a Development Environment
Python can be written and executed in various environments.
3.Learn the Basics
Familiarize yourself with the basic concepts of Python, such as variables, data types, and control flow.
4.Write Your First Program
Write a "Hello, World!" program to ensure your Python installation is functioning correctly and familiarize yourself with the syntax.
5.Understand Data Structures
Learn to create and manipulate data structures in Python to store and organize data effectively.
6.Dive into Functions
Functions in Python are used to encapsulate reusable pieces of code, so it is important to understand the concepts of parameters and return values.
7.Explore Modules and Packages
Python offers a variety of modules and packages that can be used to extend its functionality, such as math operations, file handling, and networking.
8.Practice, Practice, Practice
Reinforce your learning by practicing coding exercises and small projects.
9.Seek Learning Resources
Utilize online tutorials, video courses, and books specifically designed for Python beginners.
10.Join the Community
Engage with the Python community by participating in forums, attending local meetups, or joining online communities.
Learning Python is a continuous process, so don't be afraid to experiment and make mistakes. Practice and persistence will help you become proficient.
Installing Python on several systems (Windows, macOS, and Linux)
Installing Python on different systems is a straightforward process. Here are the general steps to install Python on Windows, macOS, and Linux:
1.Windows
Navigate to the Downloads area of the official Python website (https://www.python.org/).
Scroll down to "Python Releases for Windows" and click the "Download Python" button to get the most recent stable version.
Launch the downloaded installer and click the "Install Now" button.
Complete the installation by following the steps.
2.macOS
Go to the official Python website (https://www.python.org/) with a web browser.
Scroll down to the "Mac OS X" section on the downloads page and click on the macOS installation.
Complete the installation by following the steps.
During the installation procedure, check the box to install the "Add Python to PATH" option.
Launch a terminal window on your Linux machine.
Run the command python --version or python3 --version to see if Python is already installed.
The version number of Python will be displayed if it is already installed.
Once the installation is complete, run python to check the installation.
3.Linux
Creating a programming environment
Creating a programming environment involves choosing an IDE that provides tools and features for coding, debugging, and testing. Popular Python IDEs are available.
1.PyCharm
PyCharm is a powerful IDE with code completion, debugging, version control, and support for web development frameworks.
2.Visual Studio Code
Visual Studio Code is an IDE developed by Microsoft to enhance coding experience with IntelliSense, debugging, and extensions.
3.IDLE
IDLE is a Python IDE with features like code highlighting, interactive interpreter, and debugger, making it a good choice for beginners.
4.Spyder
Spyder is an open-source Python IDE for data analysis and scientific computing, while Jupyter Notebook/JupyterLab is an enhanced version with more features.
5.Atom
Atom is an open-source text editor that can be transformed into a powerful Python development environment.
6.Sublime Text
Sublime Text is a lightweight and versatile text editor known for its speed and efficiency.
Create and run your first Python programme
To create and run your first Python program, follow these steps:
- Open a text editor or an Integrated Development Environment (IDE) of your choice.
- Type the following code into the editor: '''python print("Hello, World!") '''
- Save the file with a .py extension, for example, 'hello_world.py'. Choose a location on your computer where you want to save the file.
- Open a terminal or command prompt on your computer.
- Navigate to the directory where the Python file is saved by using the 'cd' command.
- Run the Python file by typing'shell python hello_world.py' in the correct directory. If you have multiple versions of Python installed, use 'python3' instead.
- Experiment with Python programming by executing and displaying "Hello, World!" in the terminal.
Understanding the Basics
Understanding the basics of Python is essential for building a strong foundation in programming.
Control flow statements allow you to control the execution of your code based on conditions.
Key control flow statements in Python include if-else statements, for loops, while loops, and the use of the range function.
Understanding how to define and use functions is crucial for writing modular and efficient code.
Familiarize yourself with these data structures and their respective methods for manipulating and accessing data.
Learn how to import and use modules to leverage existing code and enhance your programs with additional features.
Variables and data types in Python
Understanding variables and data types is essential for working well with Python, including the most commonly used data types.
1.Number Formats
Integers are whole numbers without decimal points, floating-point numbers have decimal points, and complex numbers have both real and imaginary elements. Examples include x = 10, y = 3.14, and z = 2 + 3j.
2.Strings (str)
Character sequences wrapped in single quotes ('') or double quotes (""). Name = "John," for example, or message = "Hello, world!" Strings are immutable, which means they cannot be modified after they are created.
3.Boolean (bool)
This type of value represents the truth values True or False. Booleans are frequently utilised in programme decision-making and control flow. Is_raining = True, for example, or is_valid = False.
4.Lists (list)
An ordered collection of objects surrounded by square brackets ([]). Lists can include components of many data kinds and can be updated after they are created. For instance, numbers = [1, 2, 3, 4, 5] and fruits = ["apple", "banana", "orange"].
5.Tuples (tuple)
Tuples are ordered collections of objects contained in brackets (()), similar to lists. Tuples, on the other hand, are immutable, which means they cannot be changed once generated. Point = (3, 4) or colours = ("red", "green", "blue").
6.Dictionaries (dict)
Key-value pairs surrounded by curly braces (). You may obtain values from dictionaries using their related keys. Person = "name": "John", "age": 30, "city": "New York", etc.
7.Sets (set)
An unordered collection of distinct elements surrounded in curly braces (). Sets may be used to execute mathematical operations such as union, intersection, and difference. Unique_numbers = 1, 2, 3, 4, 5, for example.
Python has functions and methods for working with certain data types. To assign a value to a variable, use the equal symbol (=). For example, is_valid = True numbers = [1, 2, 3, 4, 5], x = 10, name = "John". Understanding variables and data types allows for successful storage, handling, and analysis of information in Python programmes.
Operators and expressions
Operators are symbols in Python that perform various operations on operands (values or variables). Expressions are groups of operators, variables, and values that produce a result.
Here are some of the most frequently used operators and phrases in
Python:1.
1.Arithmetic Operators
- Addition: + - Subtraction: - - Multiplication: * - Division: / - Modulus (remainder):% - Exponentiation: ** - Floor division (quotient without remainder): // Example: '''python a = 10 b = 3 result = a + b * (a - b) / a '''2.
Comparison Operators: - Equal to: == - Not equal to: !
Assignment Operators: - Assignment: = - Addition assignment: += - Subtraction assignment: -= - Multiplication assignment: *= - Division assignment: /= - Modulus assignment:%= - Exponentiation assignment: **= - Floor division assignment: //= Example: '''python x = 10
x += 5 # equivalent to x = x + 5 '''4.
2.Logical Operators
- Logical AND: and - Logical OR: or - Logical NOT: not Example: '''python x = 5 y = 10 result = (x > 0) and (y < 20) '''5.
3.Bitwise Operators
- Bitwise AND: & - Bitwise OR: | - Bitwise XOR: ^ - Bitwise NOT: ~ - Left shift: << - Right shift: >> Example: '''python x = 10 y = 5 result = x & y '''6
4.Membership Operators
- in: checks if a value exists in a sequence - not in: checks if a value does not exist in a sequence Example: '''python fruits = ['apple', 'banana', 'orange'] result = 'apple' in fruits '''7.
5.Identity Operators
- is: checks if two variables refer to the same object - is not: checks if two variables do not refer to the same object Example: '''python x = [1, 2, 3] y = [1, 2, 3] result = x is y '''These are just a few examples of operators and expressions in Python.
Control flow statements (if-else, loops)
Regulate flow statements are used in Python to regulate code execution depending on particular conditions or to repeat a block of code many times. If-else statements and loops are the most common control flow statements in Python. Here's a breakdown of each:
1. If-else statements
You may use the if-else statement to run distinct pieces of code based on a condition. The syntax is as follows:
if condition:
# code to be executed if condition is True
else:
# code to be executed if condition is False
2. Nested if-else statements
To handle several situations, nest if-else statements within each other. The inner if-else phrases are indented to do this.
Example:
x = 10
if x > 0:
print("x is positive")
else:
if x < 0:
print("x is negative")
else:
print("x is zero")
3.Elif Statement
The elif statement allows you to verify many conditions without having to layer if-else statements. It is an abbreviation for "else if". Following an initial if statement, you can have numerous elif statements.
if condition1:
# code to be executed if condition1 is True
elif condition2:
# code to be executed if condition2 is True
else:
# code to be executed if both condition1 and condition2 are False
x = 5
if x > 0:
print("x is positive")
elif x < 0:
print("x is negative")
else:
print("x is zero")
Exploring Python's Built-in Data Structures
Lists
Creating, accessing, and manipulating elements
Tuples
Immutable sequences in Python
Dictionaries
Key-value pairs for efficient data storage
Sets
Unordered collections of unique elements
Using Functions and Modules
Creating and using functions, recognising arguments and return values, import and use OOP, creating and using classes, encapsulation, inheritance, and polymorphism, handling exceptions and errors, and exploring the Python Standard Library.
Examples of useful modules and their functionalities
Python has a large number of modules that extend the capability of the core language. Here are some examples of widely used modules and their functions:
1.math
The math module contains mathematical functions and constants used in mathematical processes, such as trigonometry, logarithms, exponentiation, and rounding.
Example:
import math
x = math.sin(math.pi/2)
y = math.sqrt(25)
z = math.floor(3.7)
2.datetime:
The datetime module allows users to manipulate and format dates, times, time intervals, and timezones.
Example:
import datetime
current_time = datetime.datetime.now()
date_string = current_time.strftime("%Y-%m-%d")
3.Random
The random module generates random numbers, selects random elements from sequences, and shuffles sequences.
Example:
import random
random_number = random.randint(1, 10)
random_element = random.choice(['apple', 'banana', 'orange'])
random.shuffle([1, 2, 3, 4, 5])
4 .OS model
The os module allows users to manipulate files and directories, set environment variables, and control processes.
Example:
import os
current_directory = os.getcwd()
os.mkdir('new_directory')
file_exists = os.path.exists('file.txt')
5. Request
The requests module is used to send HTTP requests to web servers, allowing for operations such as retrieving web pages, performing API queries, and more.
Example:
import requests
response = requests.get('https://api.example.com/data')
json_data = response.json()
6. csv
The csv module allows users to interact with CSV files, read and write them, interpret them, and execute tabular data operations.
Example:
import csv
with open('data.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
7. print(row)
Python modules are available in the Python Standard Library for a variety of functions, such as database operations, networking, data processing, web development, and scientific computing. The PyPI also has third-party libraries that provide specialised functionality for certain fields.
Python for Real-World Applications
Python is widely used for a variety of real-world applications across different domains.
Here are some areas where Python is commonly applied
1.Web Development
Python's simplicity and versatility make it a popular choice for web development.
2.Data Science and Machine Learning
Python is the language of choice for data science and machine learning, with TensorFlow and PyTorch being used for deep learning.
3.Scientific Computing
Python is a popular choice for scientific computing due to its integration with popular libraries and ability to interface with other languages.
4.Automation and Scripting
Python's simplicity and ease of use make it a great language for automation and scripting tasks.
5.DevOps and Infrastructure
Python is widely used in DevOps and infrastructure automation.
6.Internet of Things (IoT)
Python is a popular choice for IoT applications due to its versatility, ease of use, and extensive ecosystem of libraries and frameworks. It is used in many domains due to its versatility, ease of use, and extensive ecosystem.
Web development with Python
Python is widely used for web development, and there are two popular frameworks that are commonly used: Django and Flask.
1.Django
Django is a high-level Python web framework that follows the Model-View-Controller (MVC) architectural pattern. It includes an ORM layer, template engine, URL routing system, security features, and an admin interface for managing data.
Example of a Django application:
# views.py
from django.shortcuts import render
def home(request):
return render(request, 'home.html')
# urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='home'),]
2.Flask
Flask is a lightweight and flexible web framework that follows the Model-View-Template (MVT) pattern. It offers essential features such as routing, request handling, and template rendering, but allows developers to choose additional libraries for specific functionality.
Example of a Flask application:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
if __name__ == '__main__':
app.run()
Django and Flask are both powerful Python web development tools, but the decision between them is influenced by project needs, complexity, and personal preferences.
Data analysis and visualization using Python libraries (NumPy, Pandas, Matplotlib)
NumPy, pandas, and Matplotlib are popular tools for data analysis and visualisation in Python, providing complete tools for working with data, calculations, and visualisation.
1.NumPy
NumPy is a core Python package for numerical computation that supports massive, multi-dimensional arrays and matrices, as well as fast array-based mathematical operations. It serves as the foundation for other Python data analysis modules.
Example:
import numpy as np
data = np.array([1, 2, 3, 4, 5])
mean = np.mean(data)
2.Pandas
Pandas is a powerful data manipulation and analysis package that provides data cleansing, filtering, aggregation, merging, data indexing, slicing, filtering, data cleansing, missing value management, and transformation functions. For example, import pandas as pd data = 'Name': 'John', 'Jane': 'Mike', 'Age': '25, 30, 35', 'City': 'New York, London, Paris'] df = pd.DataFrame(data) mean_age = df['Age'].mean('25').)
3.Matplotlib
Matplotlib is a Python toolkit for producing static, animated, and interactive visualisations, with a variety of plotting functions and NumPy and Pandas integration. Examples include import matplotlib.pyplot as plt, x = [1, 2, 3, 4, 5], y = [2, 4, 6, 8, 10], plt.plot(x, y), plt.xlabel('X'), plt.ylabel('Y'), plt.title('Sample Plot') and plt.show().
Introduction to machine learning with Python
Machine learning is the study of techniques and models that allow computers to learn from data and make predictions. Python is a popular machine learning language due to its ease of use, libraries, and community support.
1.scikit-learn
Scikit-learn is a machine learning library in Python that supports a wide range of machine learning algorithms, making it easy to integrate into existing Python data analysis workflows.
# Load the iris dataset
iris = datasets.load_iris()
X = iris.data
y = iris.target
# Split the data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a logistic regression model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions on the test set
y_pred = model.predict(X_test)
# Evaluate the model's accuracy
accuracy = accuracy_score(y_test, y_pred)
1.TensorFlow and Keras
TensorFlow is an open-source library for machine learning and deep learning, while Keras is a high-level neural networks API that simplifies the process.
Example of using TensorFlow and Keras for deep learning:
import tensorflow as tf
from tensorflow import keras
# Load and preprocess the MNIST dataset
(X_train, y_train), (X_test, y_test) = keras.datasets.mnist.load_data()
X_train = X_train / 255.0
X_test = X_test / 255.0
# Build and train a simple convolutional neural network
model = keras.models.Sequential([
keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
keras.layers.MaxPooling2D((2, 2)),
keras.layers.Flatten(),
keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
Encouragement for beginners to continue their Python learning journey
Learning Python is a great journey for beginners, as it is one of the most popular programming languages in the world. It is versatile and can be used in various domains such as web development, data science, machine learning, automation, and more. It is known for its simplicity and readability, practical and real-world applications, and career opportunities. By mastering Python, you increase your chances of landing rewarding job opportunities and advancing your career. Learning programming takes time and practice, so be patient with yourself and celebrate your progress along the way.