The definition of Java
Java is a high-level, object-oriented programming language developed by Sun Microsystems in 1995. It has platform independence, automatic memory management, and strong type checking, making it a reliable and safe language for building complex applications. It is often used for enterprise applications, Android mobile apps, and web development, with the Java-based Spring Framework being a popular choice.
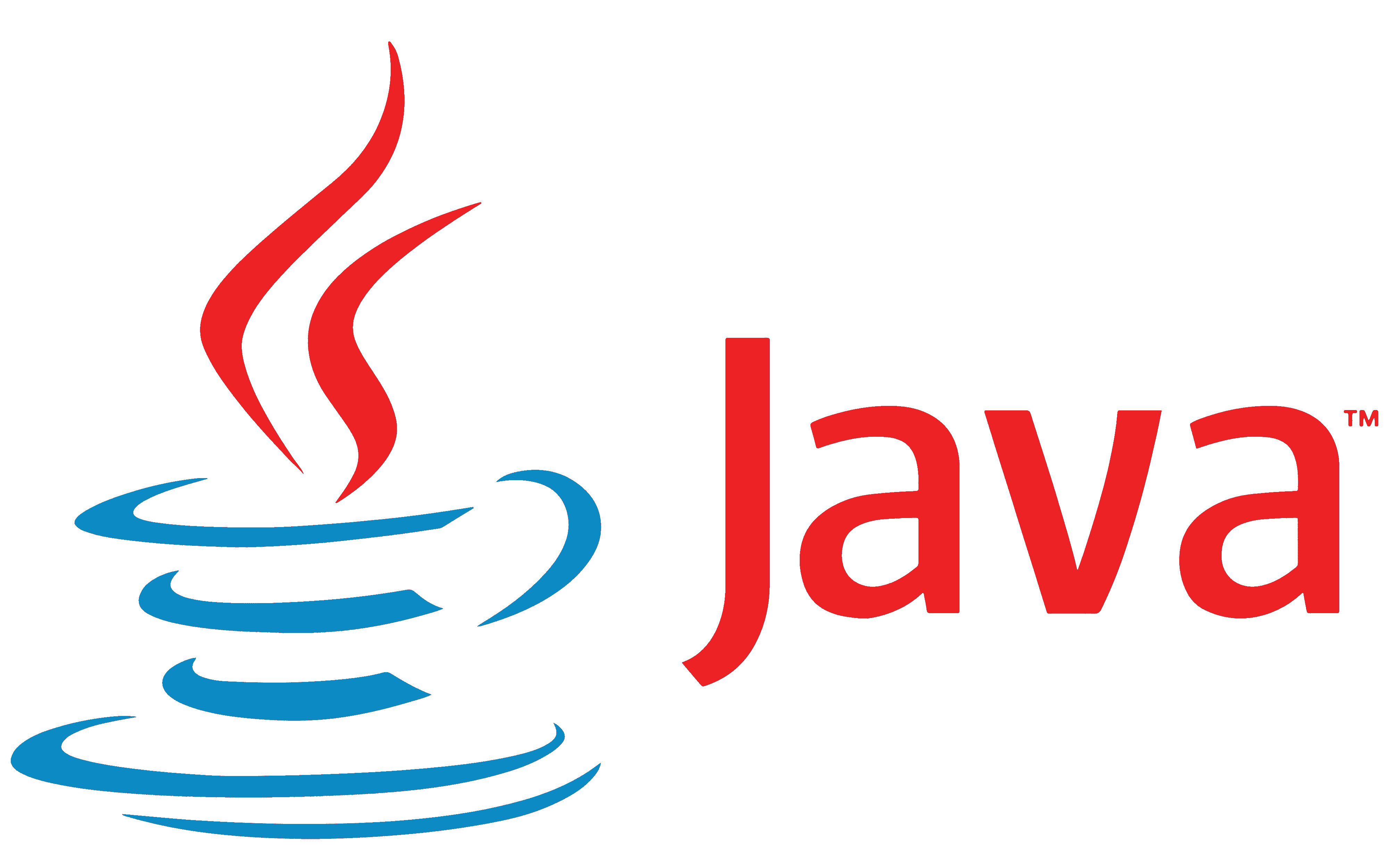
Logo of Java
Java's Brief History
Java was developed by James Gosling and Sun Microsystems in the early 1990s to provide a simpler and more reliable programming language. It quickly gained popularity due to its platform independence, writing once and running anywhere, and dynamic web applications.
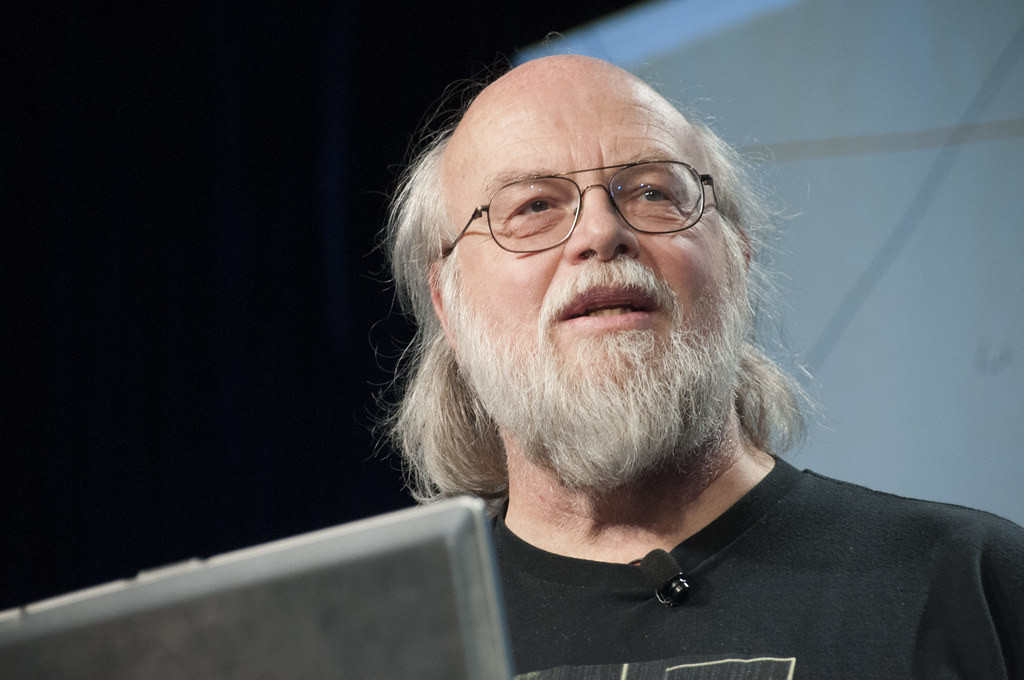
James Gosling
Java's Importance in Modern Computing
Java is an extremely important language in modern computing due to its versatility, reliability, and popularity.
Here are some of the key reasons why Java is so important in modern computing:
Platform Independence
Java's ability to run on any platform with a Java Virtual Machine (JVM) is essential for developing applications that need to run on different operating systems and devices.
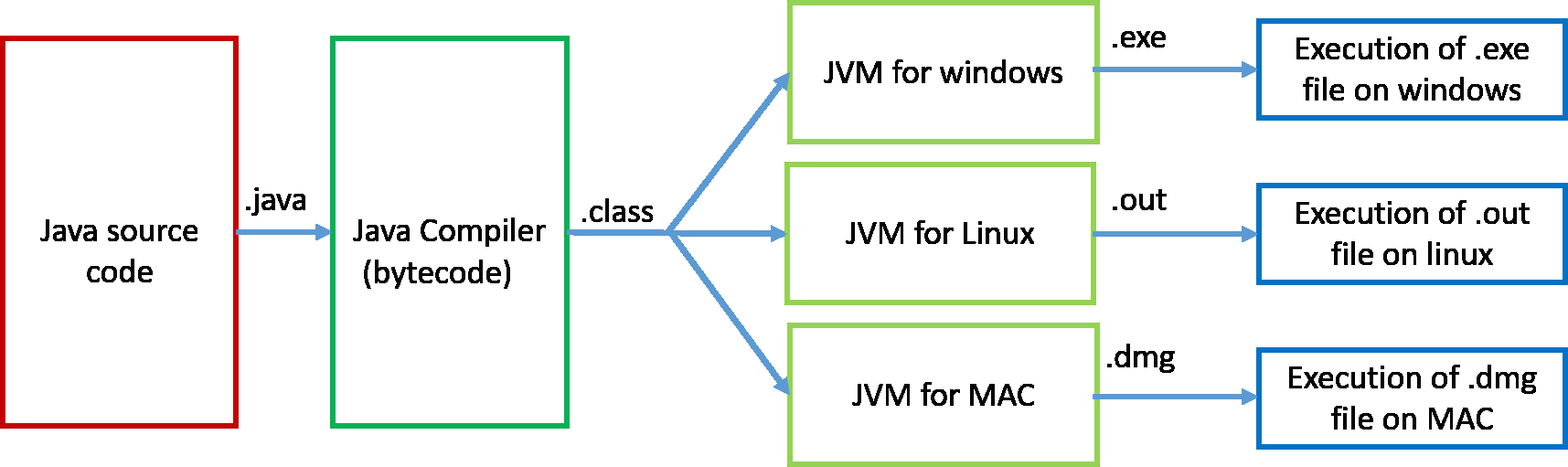
Platform Independence
Enterprise Applications
Java is a powerful language for building modern web applications due to its scalability, robustness, and support for web technologies.
Big Data
Java is used for big data processing due to its ability to handle large volumes of data and its support for multithreading and concurrency.
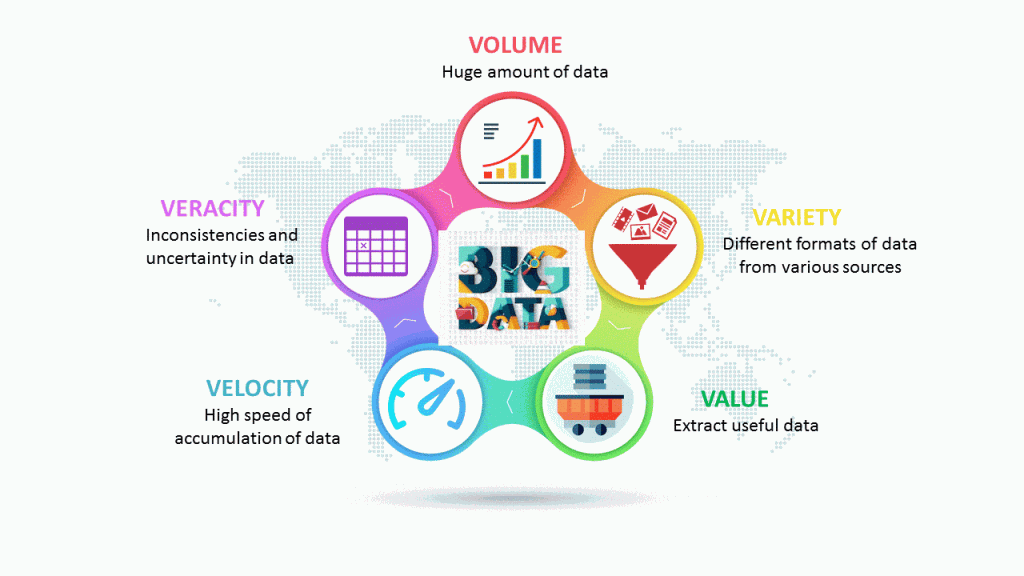
Big Data in Java
Java Fundamentals
Java is an object-oriented programming language used for a variety of applications, with its syntax based on the C programming language. Curly braces and semicolons are used to define blocks of code and terminate statements.
Object-Oriented Programming
Java is an object-oriented programming language, meaning that it focuses on defining and manipulating objects. This allows for greater flexibility and modularity in the code.
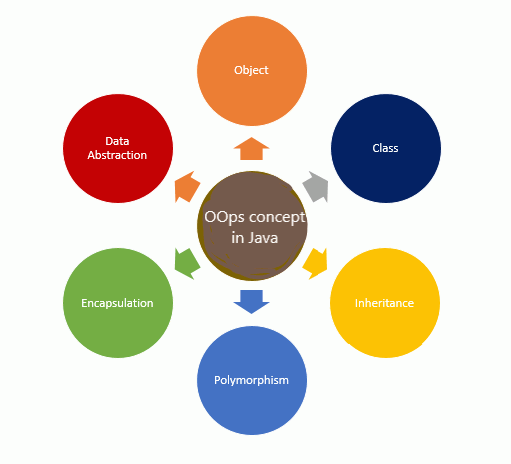
Object-Oriented Programming
Exception Handling
Java has a built-in exception-handling mechanism that allows developers to handle errors and exceptions that occur during program execution.
Garbage Collection
Java has automatic garbage collection, meaning that it automatically manages memory allocation and deallocation for objects.
Java Virtual Machine (JVM)
Java runs on the Java Virtual Machine (JVM), which provides a layer of abstraction between the Java code and the underlying hardware. Java is an object-oriented programming language with a syntax based on C, support for inheritance and interfaces, automatic garbage collection, and a platform-independent architecture based on the JVM.
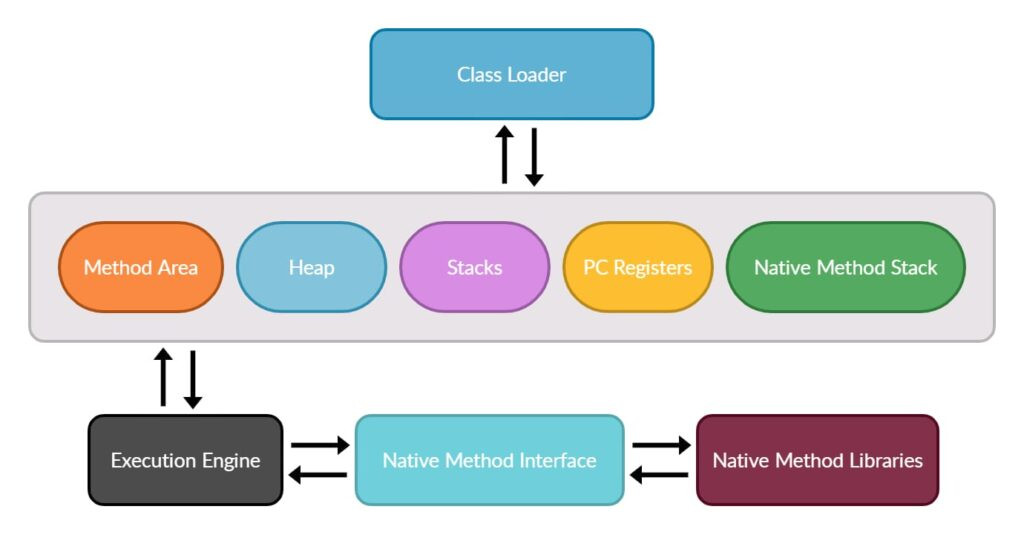
Java Virtual Machine (JVM)
Variables and syntax
Variables in Java are used to store data values that can be used in a program. They must be declared with a specific data type, initialized with a default value, and have a scope that defines where they can be accessed in the program. Variables declared outside a block of code have global scope and can be accessed throughout the program.
Operators and data types
Operators and data types are two important concepts in Java that are used to manipulate data values in a program. Operators are used to perform mathematical, logical, and relational operations, while data types specify the type of data that a variable can hold. Type casting is used to convert a value from one data type to another, while strings are a special type of reference data type used to represent text.
Advanced Java
Advanced Java is a set of technologies and APIs used to develop complex, scalable, and distributed applications in Java. It includes topics not covered in the core Java programming language, such as Servlets, JSP, JPA, EJB, and JDBC. It also provides powerful tools and frameworks to develop dynamic, data-driven web applications, database connectivity, Object-Relational Mapping, Enterprise Applications, and Security. Advanced Java is a vast and complex topic that requires a deep understanding of various Java technologies and frameworks and is essential for developers who want to build complex, scalable, and secure applications in Java.
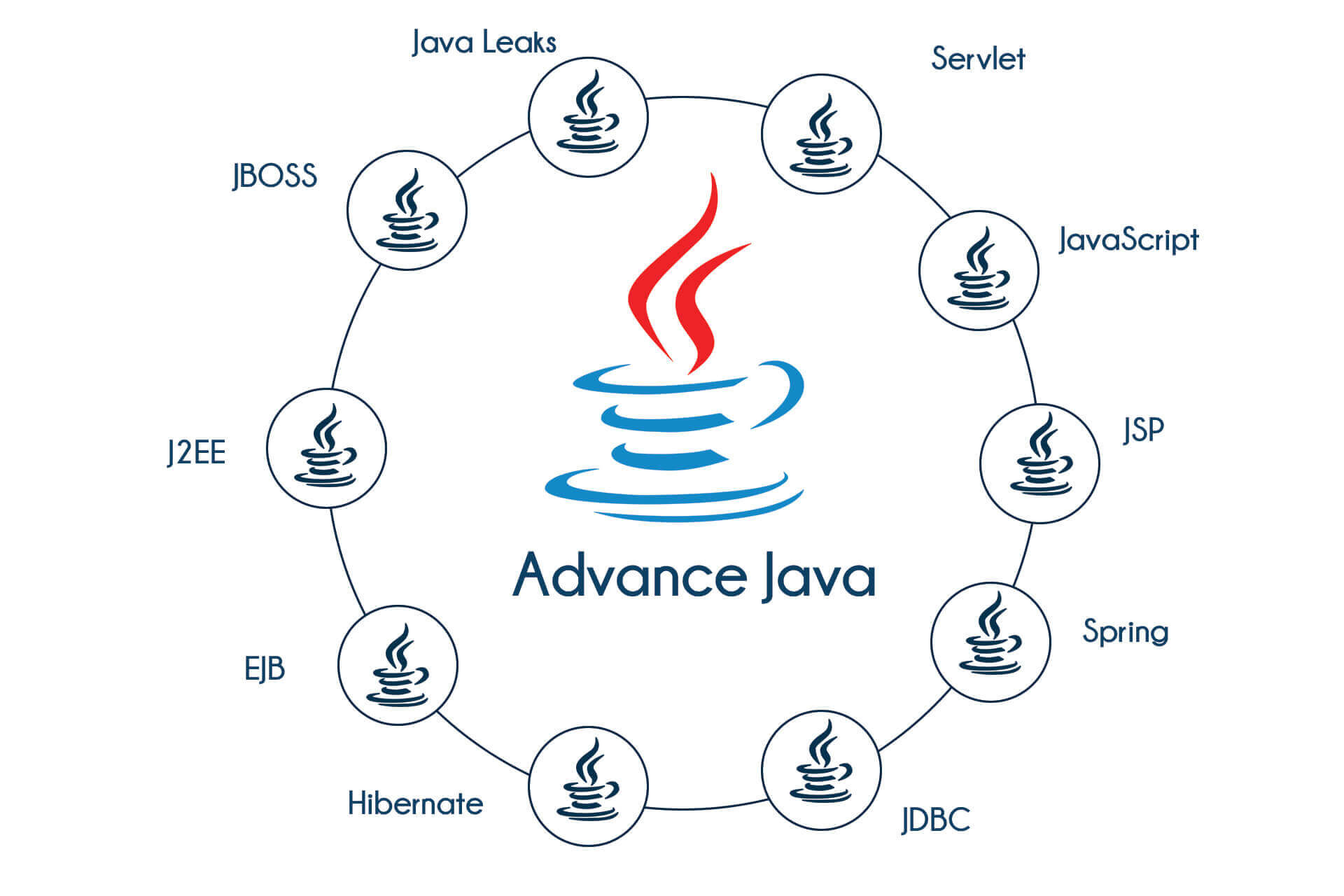
Advanced Java
Polymorphism and inherited traits
Polymorphism and inherited traits are both important concepts in genetics, as they help to explain the diversity of traits observed in living organisms. Polymorphism is caused by differences in the genes that control the expression of a trait, while inherited traits are passed down through genetic material.
Handling of exceptions
Try-catch blocks are code constructs that allow developers to handle runtime errors and exceptions during the execution of a JavaScript program. If an exception is thrown, the code execution is stopped and the catch block is executed.
Multithreading Networking IV. Common Java Applications
Java is a versatile language that can be used in a wide range of applications, from web and enterprise development to gaming, big data processing, and mobile app development. Its multithreading and networking capabilities make it a versatile language.
Development of desktop applications
JavaScript is a popular language for web development, but can also be used to build desktop applications. There are several frameworks and tools available to help developers create cross-platform desktop applications, such as Electron, NW.js, Node-webkit, and React Native.
Additionally, Node.js, Angular, React, and Express.js are web application frameworks for Node.js, allowing developers to leverage their existing JavaScript skills and build scalable, high-performance, and maintainable enterprise applications.
Development of Android mobile applications
JavaScript is used for web development and Android mobile applications, and popular frameworks and tools such as React Native, NativeScript, PhoneGap, and Cordova allow developers to leverage their existing JavaScript skills.
The use of big data with machine learning
JavaScript is a versatile language that can be used in various areas of technology, including big data and machine learning. It can be used for data visualization, data processing, machine learning, natural language processing, and predictive analytics. Its versatility and accessibility make it a powerful tool for developers working in the big data and machine learning space.
Java Best Practices
Here are some best practices for Java development: Use meaningful variable and method names: Use descriptive names for variables and methods that indicate their purpose.
Follow coding conventions
Adhere to established coding conventions, such as the Java Naming Conventions and the Java Code Conventions. This makes the code more consistent and easier to read for other developers.
1. Write modular code
Divide your code into small, reusable modules that perform specific tasks.
2.Use comments
Include comments in your code to explain the purpose of the code and how it works.
3.Test your code
Test your code thoroughly using unit tests, integration tests, and other testing techniques.
4.Optimize performance
Optimize the performance of your code by avoiding unnecessary loops, minimizing object creation, and using appropriate data structures. This helps to improve the speed and efficiency of the application.
5.Use design patterns
Use established design patterns, such as the Singleton pattern and the Factory pattern, to create scalable and maintainable code.
6.Use version control
Use a version control system, such as Git or SVN, to manage changes to your code.
7.Follow security best practices
Follow security best practices, such as input validation and encryption, to prevent security vulnerabilities in your application.
Organization and readability of code
Organizing and making your code readable is essential for making it easy to understand, maintain, and debug.
Here are some tips for organizing and improving the readability of your code:
1.Use meaningful names
Use descriptive names for variables, functions, and classes that convey their purpose.
2.Break down code into smaller functions
Break down complex code into smaller functions that perform a specific task.
3.Use whitespace and indentation
Use whitespace and indentation to make your code more readable.
4.Organize code into modules or packages
Organize your code into modules or packages based on functionality.
5.Use design patterns
Use established patterns to make your code more modular and reusable. This makes it easier to understand and modify your code in the future.
6.Write clear and concise code
Write code that is clear and concise.
7.Test your code
Test your code thoroughly to ensure it works as expected.
Java Ecosystem VI
JVM (Java Virtual Machine)
The Java Virtual Machine (JVM) and Java Development Kit (JDK) are important components of the Java ecosystem.
JDK (Java Development Kit)
The JVM is an abstract computing machine that provides the runtime environment for Java programs.
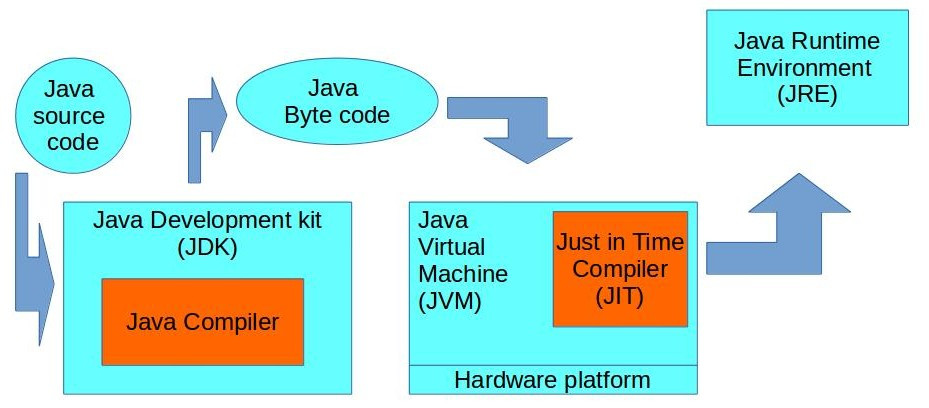
JDK (Java Development Kit)
The Java Virtual Machine (JVM) is a programming language that interprets Java bytecode and translates it into machine-specific instructions. The JDK is a software development kit that includes tools and libraries for developing Java applications. It includes the Java compiler, debugger, documentation generator, and libraries and APIs that make it easier to develop Java applications. Together, the JVM and JDK form the core of the Java ecosystem, enabling developers to write robust, secure, and high-performance Java applications for a variety of platforms and devices.
Java SE (Java Standard Edition)
Java SE (Java Standard Edition) is a platform that provides a set of APIs, tools, and libraries for developing Java applications that run on desktops, servers, and other embedded devices. It provides the core features of the Java programming language, including language syntax, libraries, and JVM (Java Virtual Machine). Java SE includes a range of APIs that provide features such as networking, I/O, threading, graphics, and security. It also includes a range of tools and utilities that make it easier to develop, test, and deploy Java applications. Java EE (Java Enterprise Edition) is an acronym for Java Enterprise Edition.
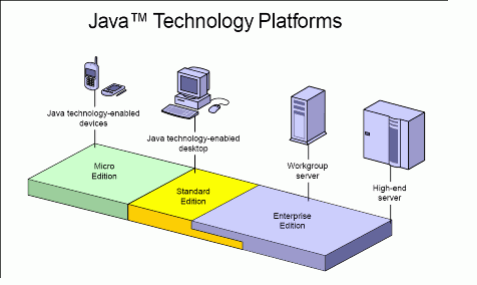
JavaSE-EE-ME-compared
Java's Future
Java is one of the most popular programming languages today, and its future looks bright. Key trends and developments that are likely to shape the future of Java include the expansion of the Java ecosystem, the continued evolution of the language, a focus on performance and scalability, security, and the adoption of new technologies. These trends are likely to continue to shape the development of Java in the years to come, with a strong and growing ecosystem, the continued evolution of the language, and a focus on performance, scalability, and security.
Current developments and trends
Java 17 was released in September 2021, with several new features and enhancements. Microservices and cloud-native applications have gained popularity as a way to build highly scalable and modular applications. Reactive programming is an approach to building applications that are responsive, resilient, and elastic. Machine learning and AI drive the development of new libraries and frameworks for building intelligent Java applications. Functional programming has gained popularity as a way to write more concise and expressive code.
Kotlin is a programming language that runs on the Java Virtual Machine and is widely used in Android app development. Java frameworks and libraries to keep an eye on include Spring Framework, Quarkus, Hibernate, Apache Kafka, and Micronaut. These frameworks and libraries are likely to have a significant impact on the software development business, as they provide tools for building scalable, modular, and cloud-native applications. Developers need to stay up-to-date with the latest trends and technologies to remain competitive.
Recap of Java's Relevance and Adaptability
Java's relevance and adaptability are key factors that have contributed to its longevity and continued popularity in the software development industry. These include platform independence, a large developer community, strong enterprise support, evolving language features, and adaptability to new technologies. These features have enabled Java to maintain its position as one of the most popular programming languages in the world and are likely to remain a key player in the software development industry for many years to come.
Encouragement to keep studying and exploring the language
Java is a language that offers a lot of opportunities for personal and professional growth. It is a versatile language that can be used to build a wide range of applications, and it has a large and active developer community that provides support, resources, and tools for Java developers. Keeping up-to-date with the latest Java releases will enable you to take advantage of these new features and enhance your skills.